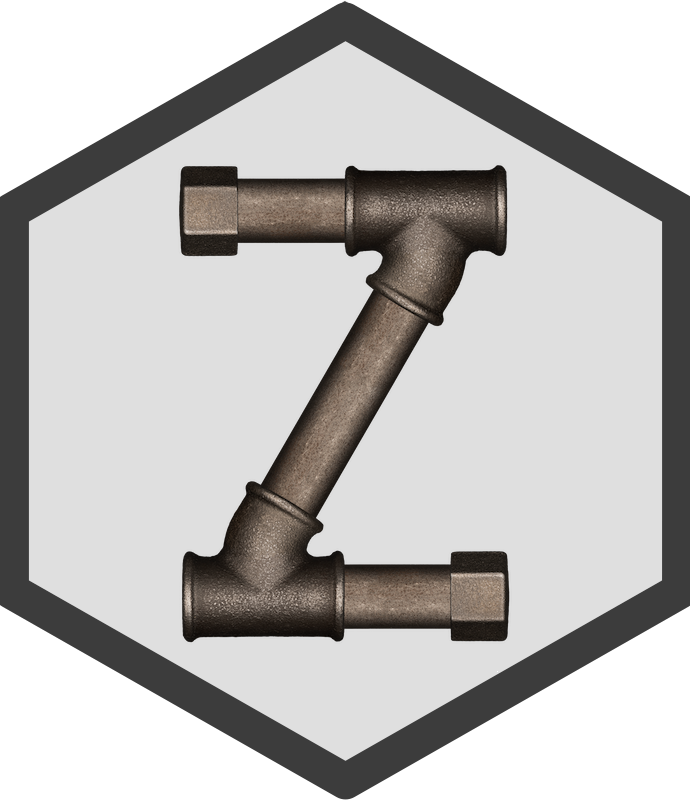
Create a pipe-friendly version of a function
Source:R/base_a_zfunction.R
, R/base_b_zfold.R
, R/base_c_zfitter.R
zfunction.Rd
These functions all serve the role of rearranging the arguments of other functions, in order to create pipe-friendly versions.
zfunction()
rearranges the arguments of any function moving the specified
argument to the front of the list, so that this argument becomes the
recipient of piping. It returns a copy of the input function, that is
identical to the original except for the order of the arguments.
zfold()
creates a pipe-friendly version of a function of the standard
format by creating a fold (or wrapper) around it with the parameters
reordered. Compared to using zfunction()
, which makes a copy of the
original function with rearranged the parameters, this creates a wrapper that
in turn will call the original function with all passed parameters. This is
good for making pipe-friendly versions of S3
generics, whereas rearranging
parameters directly will break the S3
dispatch mechanism.
zfitter()
creates a pipe-friendly version of a fitting function of the
standard format –– that is a function with a formula
parameter followed by
a data
parameter. It also shortens very long data names (longer than 32
characters by default), which otherwise are a nuisance when the data comes
from the pipe, because the pipeline gets converted to a very long function
call.
Arguments
- fun
The function to adapt (for
zfitter()
this should be a fitting function that takesformula
anddata
parameters). The name should not be quoted, rather, the actual function should be passed (prefixed with package if needed).- x
The name of the argument that should be moved to the front of the argument list. Can be passed with or without quotes, and is processed using non-standard evaluation unless surrounded with curlies, as in
{value}
, see details below.- x_not_found
How to handle the case where the value of
x
is not the name of a parameter infun
. Iferror
, abort the function. Ifok
, prepend the value to the existing parameter list. This can be useful if looking to pipe data into a parameter that is hidden by a...
.
Details
The x
parameter is processed using non-standard evaluation, which can be
disabled using curly brackets. In other words, the following are all
equivalent, and return a file renaming function with the to
parameter as
the first one:
zfunction(file.rename, to)
zfunction(file.rename, "to")
param_name <- "to"; zfunction(file.rename, {param_name})
Examples
# A a grep function with x as first param is often useful
zgrep <- zfunction(grep, x)
carnames <- rownames(mtcars)
grep("ll", carnames, value=TRUE)
#> [1] "Cadillac Fleetwood" "Toyota Corolla" "Dodge Challenger"
zgrep(carnames, "ll", value=TRUE)
#> [1] "Cadillac Fleetwood" "Toyota Corolla" "Dodge Challenger"
# zfunction() is the best approach to wrapping functions such as
# `pls::plsr()` that hide the data parameter behind the `...`.
if (requireNamespace("pls")) {
zplsr <- zfunction(pls::plsr, data, x_not_found = "ok")
zplsr(cars, dist ~ speed)
}
#> Partial least squares regression, fitted with the kernel algorithm.
#> Call:
#> plsr(formula = dist ~ speed, data = cars)
# Curly {x} handling: These are all equivalent
param_name <- "to";
f1 <- zfunction(file.rename, to)
f2 <- zfunction(file.rename, "to")
f3 <- zfunction(file.rename, {param_name})
# Using zfold() to create a grep() wrapper with the desired arg order
zgrep <- zfold(grep, x)
carnames <- rownames(mtcars)
grep("ll", carnames, value=TRUE)
#> [1] "Cadillac Fleetwood" "Toyota Corolla" "Dodge Challenger"
zgrep(carnames, "ll", value=TRUE)
#> [1] "Cadillac Fleetwood" "Toyota Corolla" "Dodge Challenger"
# Using zfitter to wrap around a fitting function
# (this is the actual way zlm_robust is defined in this package)
if (requireNamespace("estimatr", quietly = TRUE)) {
zlm_robust <- zfitter(estimatr::lm_robust)
zlm_robust(cars, speed~dist)
# The resulting function works well the native pipe ...
if ( getRversion() >= "4.1.0" ) {
cars |> zlm_robust( speed ~ dist )
}
}
#> Estimate Std. Error t value Pr(>|t|) CI Lower CI Upper DF
#> (Intercept) 8.2839056 0.90884644 9.114747 4.810799e-12 6.4565474 10.1112639 48
#> dist 0.1655676 0.02004456 8.259977 8.937493e-11 0.1252653 0.2058699 48
# ... or with dplyr
if ( require("dplyr", warn.conflicts=FALSE) ) {
# Pipe cars dataset into zlm_robust for fitting
cars %>% zlm_robust( speed ~ dist )
# Process iris with filter() before piping. Print a summary()
# of the fitted model using zprint() before assigning the
# model itself (not the summary) to m.
m <- iris %>%
dplyr::filter(Species=="setosa") %>%
zlm_robust(Sepal.Length ~ Sepal.Width + Petal.Width) %>%
zprint(summary)
}
#> Loading required package: dplyr
#>
#> Call:
#> lm_robust(formula = Sepal.Length ~ Sepal.Width + Petal.Width,
#> data = .)
#>
#> Standard error type: HC2
#>
#> Coefficients:
#> Estimate Std. Error t value Pr(>|t|) CI Lower CI Upper DF
#> (Intercept) 2.6300 0.28845 9.118 5.785e-12 2.0497 3.2103 47
#> Sepal.Width 0.6664 0.08766 7.602 1.006e-09 0.4901 0.8428 47
#> Petal.Width 0.3723 0.28977 1.285 2.052e-01 -0.2107 0.9552 47
#>
#> Multiple R-squared: 0.5631 , Adjusted R-squared: 0.5445
#> F-statistic: 37 on 2 and 47 DF, p-value: 2.23e-10